PlayFabを使うと、簡単にランキング機能を作ることが出来ます。実装には次のリンクを参考に作成しました。
https://playfab-master.com/playfab-ranking
今回作る内容
今回の実装ではランキング機能を作る上で必要になるダッシュボードへの設定や、そのデータの登録・取得方法を解説します。UIに反映する部分までは行いません。(表示反映までは配信などで行っていますので、見たい方は遊びに来てください)
出来上がり
下図ではUIまで作成しています。ランキングのパラメータを取得することでランキング表示を作ることも可能です。
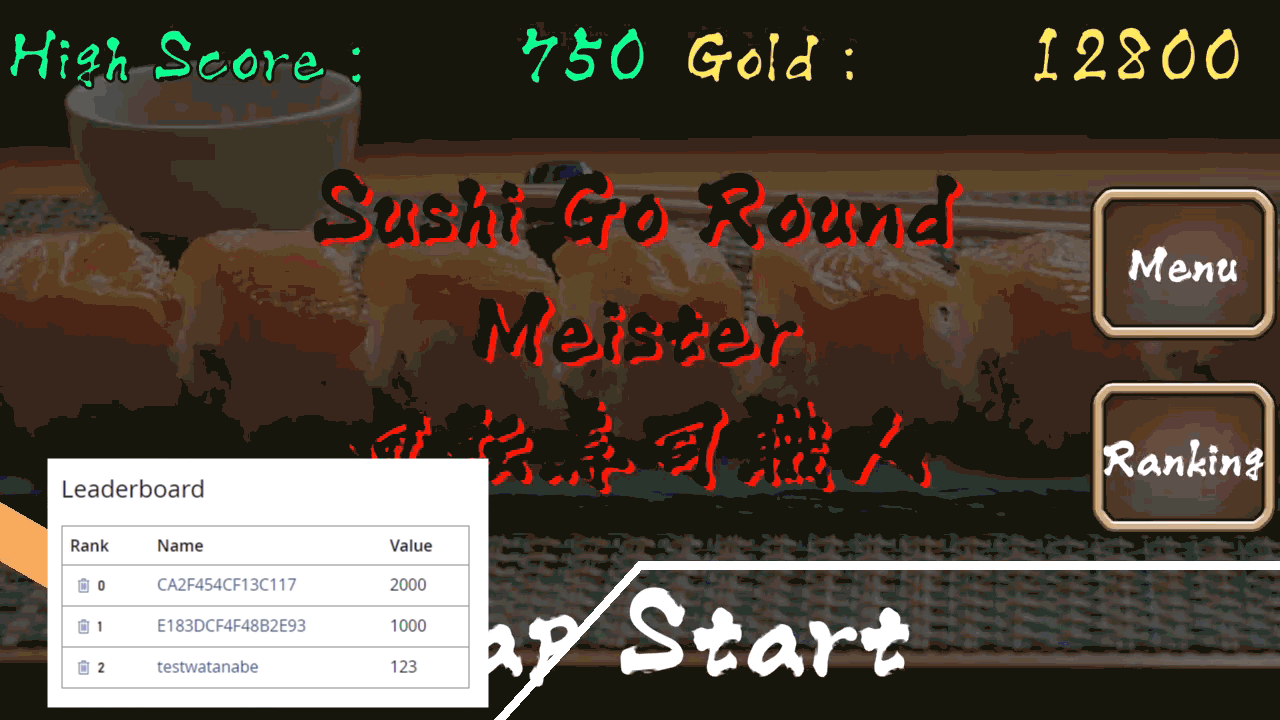
設計方針や出てくる内容
ランキングを作る上で必要になる処理など。
- ダッシュボードでの設定
- クライアントから更新できるようにする処理
- Leaderboards(ランキング)の追加
- プログラム実装
- スコアを送信する処理
- ランキングを取得する処理
その他、補足的にできるようになっておきたいこと
- 必須項目
- PlayFabへのログイン機能
- できると嬉しい
- ランキング表示のUIを作成
PlayFabへのログインが必須になります。
PlayFabの設定を行う
では作業開始しましょう。PlayFabの管理画面に移動して、ランキングを作りたいゲームに設定を加えますよ!
クライアント側からの更新を行える設定を追加
今回はサーバーはなしで、クライアントからの更新を行えるようにしたいと思います。実際のゲーム開発だとサーバー側の作成が必要になるケースもありますが、こういった部分をすっとばせるのがPlayFabのいいところですね。下図では私のゲームの「回転寿司職人」というゲームにランキングを付け加えるとして進めます。
まずはスタジオ・タイトル画面から編集したい(ランキングを追加したい)ゲームを選択します。
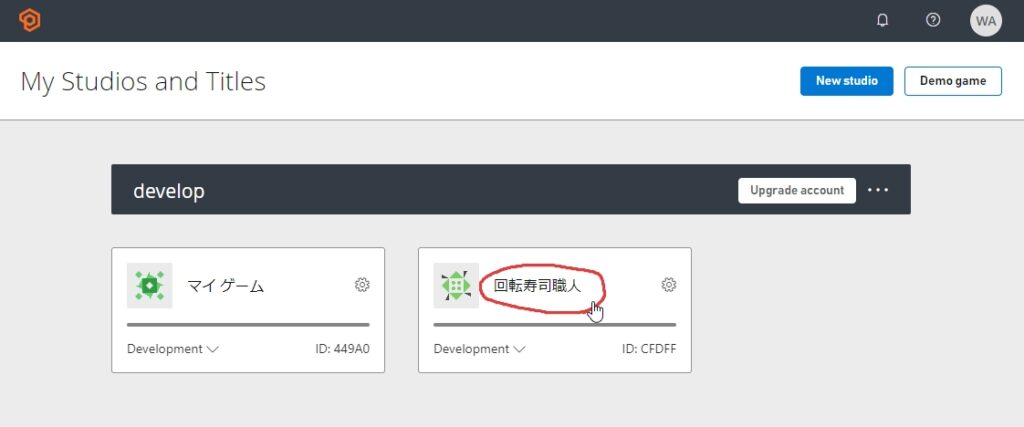
編集したいゲームの画面に移動したら、左上の歯車マーク>Title settingsを選択します。
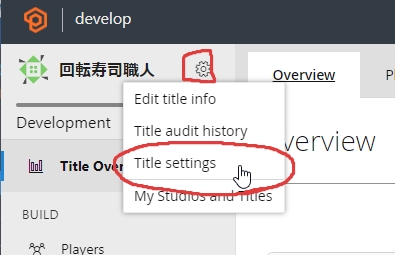
次のページに移動したら、API Featuresを選択して、画面下の方へ移動
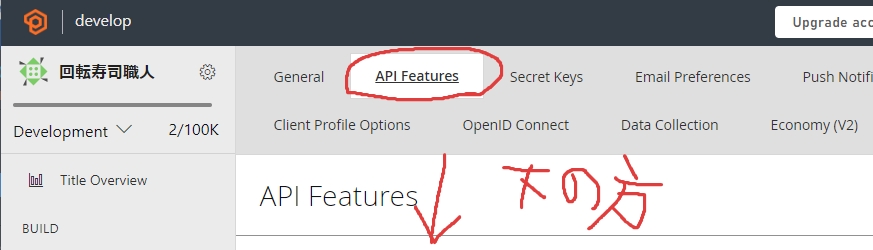
ENABLE API FEATURES>Allow client to post player statisticsにチェックを入れてSaveボタンを押して保存します。
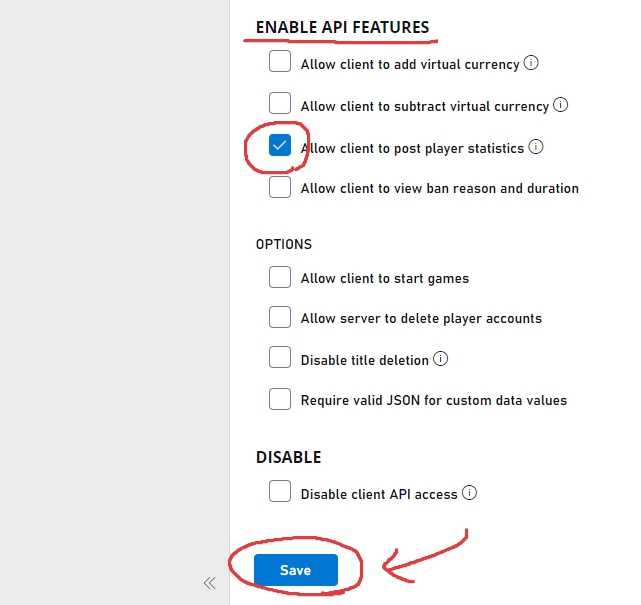
Leaderboards(ランキング)を作成
PlayFabのゲーム個別ページの左側、Leaderboards(ランキング)を選択します。
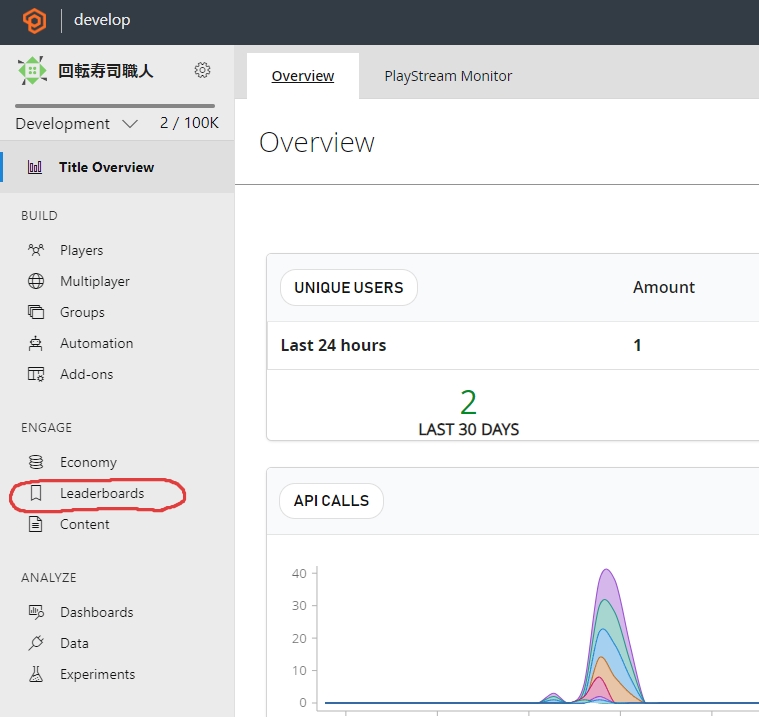
未作成の場合は下図のような画面になります。「New leaderboad」ボタンを押してください。
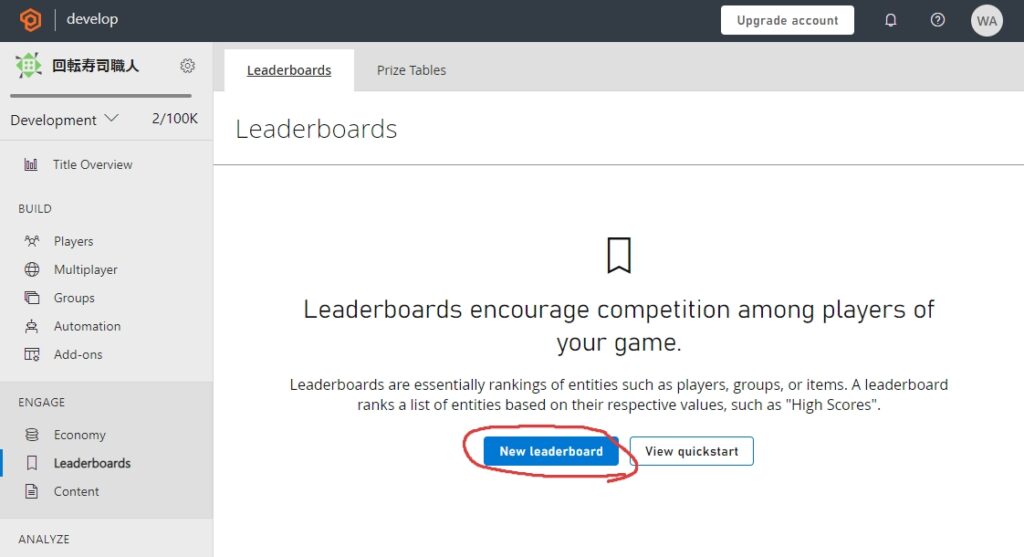
次の項目を入力してSaveボタンを押して作成されます。今回はハイスコア用のランキングを作ることを想定
項目 | 設定 | 今回 |
---|---|---|
Statistic name | ランキングの名前 プログラムでも使うので英字で作った方がいいかも | HighScore |
Reset frequency | どの期間でで集計するか 手動・1時間ごと・1日ごと・1週間ごと・一ヶ月ごと | 手動 Manually |
Aggregation method | どの値を利用するか 一番最後の・最低値・最大値・加算する | 最大値 Maximum |
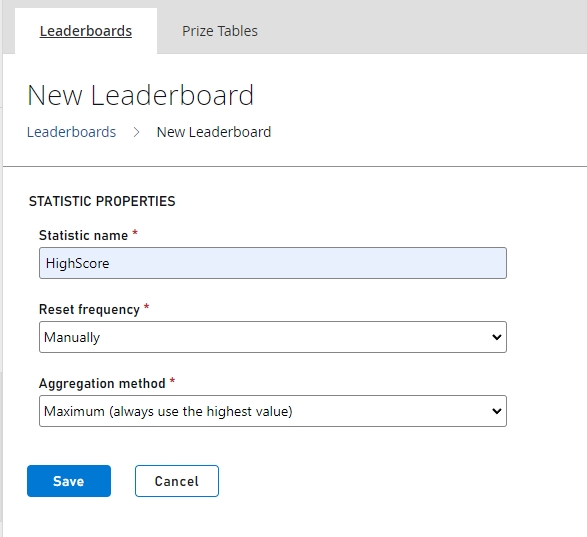
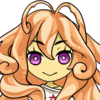
1週間の累計獲得ポイントランキングとかを作りたいなら
Weekly – Sumとかですね。ゲームによっていろいろ変えられそう。
この表示までたどり着けたらひとまず管理ページの設定は終了。
ランキングの登録・取得
では、プログラム書いてランキングに登録したり、データの取得をしてみたいと思います。プログラムはPlayFabのログインを行った作業の延長線で行います。
ランキングの登録
ランキング登録できるのはログインが完了した後に出来ます。今回は「HighScore」というLeaderboardに対して登録を行います。
using System.Collections.Generic;
using UnityEngine;
using PlayFab.ClientModels;
using PlayFab;
public class PlayFabLogin : MonoBehaviour
{
private void OnEnable()
{
PlayFabAuthService.OnLoginSuccess += PlayFabAuthService_OnLoginSuccess;
PlayFabAuthService.OnPlayFabError += PlayFabAuthService_OnPlayFabError;
}
private void OnDisable()
{
PlayFabAuthService.OnLoginSuccess -= PlayFabAuthService_OnLoginSuccess;
PlayFabAuthService.OnPlayFabError -= PlayFabAuthService_OnPlayFabError;
}
private void PlayFabAuthService_OnLoginSuccess(LoginResult success)
{
Debug.Log("ログイン成功");
SubmitScore(123);
}
private void PlayFabAuthService_OnPlayFabError(PlayFabError error)
{
Debug.Log("ログイン失敗");
}
void Start()
{
PlayFabAuthService.Instance.Authenticate(Authtypes.Silent);
}
public void SubmitScore(int playerScore)
{
PlayFabClientAPI.UpdatePlayerStatistics(new UpdatePlayerStatisticsRequest
{
Statistics = new List<StatisticUpdate>
{
new StatisticUpdate
{
StatisticName = "HighScore",
Value = playerScore
}
}
}, result =>
{
Debug.Log($"スコア {playerScore} 送信完了!");
}, error =>
{
Debug.Log(error.GenerateErrorReport());
});
}
}
上記プログラムを更新し、ゲームを動かすとログイン成功後に123というスコアを送信します。
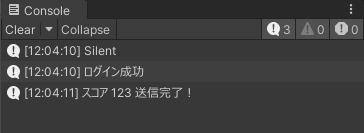
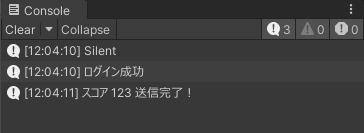
確認出来たらダッシュボードでも確認出来ます。
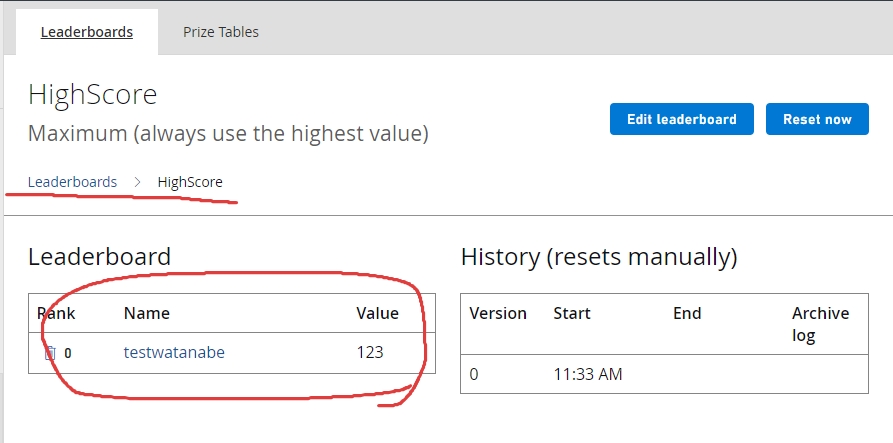
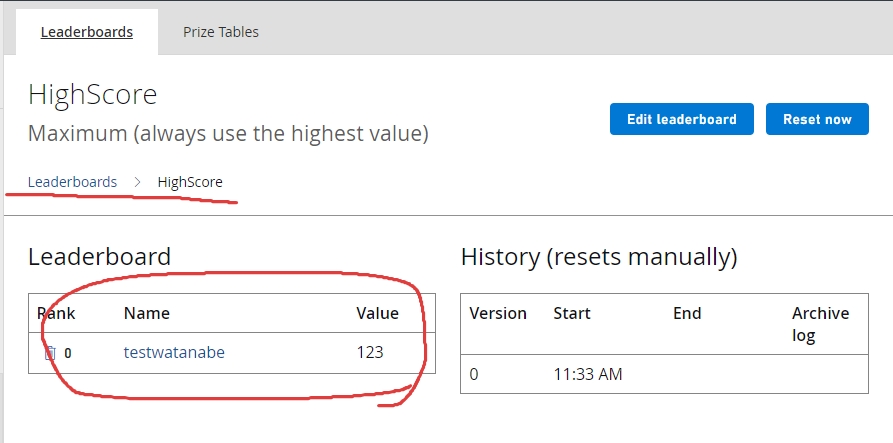
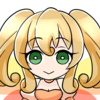
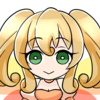
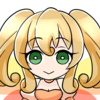
Nameに表示されるのはユーザーデータのDispalyNameになります。未登録の場合は変なIDになってるはず。。。
ランキングの取得
ランキングを取得する場合も同様にLeaderboardsに登録している名前を利用します。我々の場合は 「HighScore」ですね。先程の登録処理は一旦コメントアウトし、取得するメソッドを作成し、ログイン後に実行してみたいと思います。ソースコードは今回これで終了。全文載せておきます。
using System.Collections.Generic;
using UnityEngine;
using PlayFab.ClientModels;
using PlayFab;
public class PlayFabLogin : MonoBehaviour
{
private void OnEnable()
{
PlayFabAuthService.OnLoginSuccess += PlayFabAuthService_OnLoginSuccess;
PlayFabAuthService.OnPlayFabError += PlayFabAuthService_OnPlayFabError;
}
private void OnDisable()
{
PlayFabAuthService.OnLoginSuccess -= PlayFabAuthService_OnLoginSuccess;
PlayFabAuthService.OnPlayFabError -= PlayFabAuthService_OnPlayFabError;
}
private void PlayFabAuthService_OnLoginSuccess(LoginResult success)
{
Debug.Log("ログイン成功");
//SubmitScore(123);
GetLeaderboard();
}
private void PlayFabAuthService_OnPlayFabError(PlayFabError error)
{
Debug.Log("ログイン失敗");
}
void Start()
{
PlayFabAuthService.Instance.Authenticate(Authtypes.Silent);
}
public void SubmitScore(int playerScore)
{
PlayFabClientAPI.UpdatePlayerStatistics(new UpdatePlayerStatisticsRequest
{
Statistics = new List<StatisticUpdate>
{
new StatisticUpdate
{
StatisticName = "HighScore",
Value = playerScore
}
}
}, result =>
{
Debug.Log($"スコア {playerScore} 送信完了!");
}, error =>
{
Debug.Log(error.GenerateErrorReport());
});
}
public void GetLeaderboard()
{
PlayFabClientAPI.GetLeaderboard(new GetLeaderboardRequest
{
StatisticName = "HighScore"
}, result =>
{
foreach (var item in result.Leaderboard)
{
string displayName = item.DisplayName;
if (displayName == null)
{
displayName = "NoName";
}
Debug.Log($"{item.Position + 1}位:{displayName} " + $"スコア {item.StatValue}");
}
}, error =>
{
Debug.Log(error.GenerateErrorReport());
});
}
}
実行結果
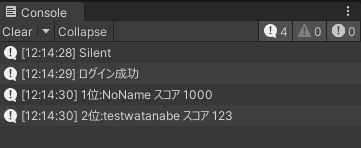
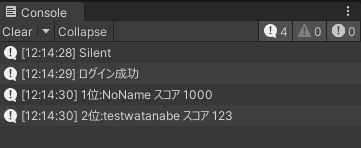
こっそり他の人のデータを増やしてます。
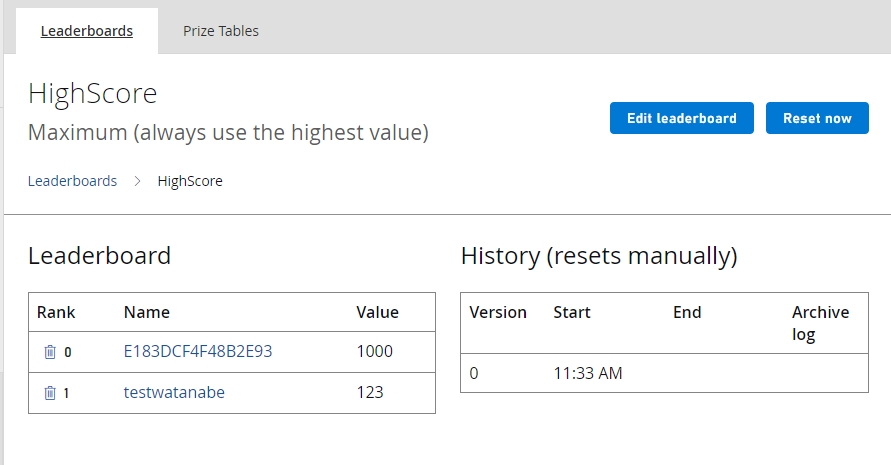
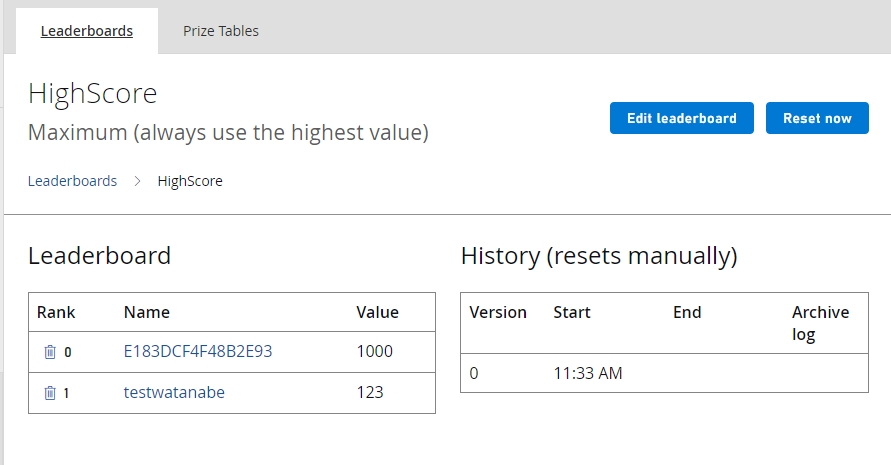
あとがき
データの取得ができた後は、foreach文の中でランキング登録されている人の名前やスコアを反映したバナー表示をしてあげることで、ランキングの表示ができるようになります。