ゲームのが一通り機能してきました。あとはスコアを表示してゲームに目標を持たせましょう。
目次
各フルーツに得点を設定する
フルーツは、進化した時に得点します。スクリプトで変数を追加し、プレファブ側で設定を行います。
スクリプトで得点の追加とイベント追加
スイカゲームで得点を行うタイミングは進化を行うタイミングです。得点時にはstaticなイベントを投げます。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Events;
public enum FRUITS_TYPE
{
さくらんぼ = 0,
いちご,
ぶどう,
オレンジ,
かき,
りんご,
なし,
もも,
パイナップル,
メロン,
すいか,
}
public class Fruits : MonoBehaviour
{
public FRUITS_TYPE fruitsType;
private static int fruits_serial = 0;
private int my_serial;
public bool isDestroyed = false;
[SerializeField] private Fruits nextFruitsPrefab;
[SerializeField] private int score;
public static UnityEvent<int> OnScoreAdded = new UnityEvent<int>();
private void Awake()
{
my_serial = fruits_serial;
fruits_serial++;
}
private void OnCollisionEnter2D(Collision2D other)
{
if (isDestroyed)
{
return;
}
if (other.gameObject.TryGetComponent(out Fruits otherFruits))
{
if (otherFruits.fruitsType == fruitsType)
{
if (nextFruitsPrefab != null && my_serial < otherFruits.my_serial)
{
OnScoreAdded.Invoke(score);
isDestroyed = true;
otherFruits.isDestroyed = true;
Destroy(gameObject);
Destroy(other.gameObject);
if (nextFruitsPrefab == null)
{
return;
}
Vector3 center = (transform.position + other.transform.position) / 2;
Quaternion rotation = Quaternion.Lerp(transform.rotation, other.transform.rotation, 0.5f);
Fruits next = Instantiate(nextFruitsPrefab, center, rotation);
// 2つの速度の平均をとる
Rigidbody2D nextRb = next.GetComponent<Rigidbody2D>();
Vector3 velocity = (GetComponent<Rigidbody2D>().velocity + other.gameObject.GetComponent<Rigidbody2D>().velocity) / 2;
nextRb.velocity = velocity;
float angularVelocity = (GetComponent<Rigidbody2D>().angularVelocity + other.gameObject.GetComponent<Rigidbody2D>().angularVelocity) / 2;
nextRb.angularVelocity = angularVelocity;
}
}
}
}
}
プレファブにスコアを設定
スクリプトを更新すると、各フルーツのプレファブにスコアが設定できるようになります。下に各フルーツに参考スコアを載せておきます。好きに設定して問題ありませんが、大きいフルーツほど高いスコアにしておくと良いですね。
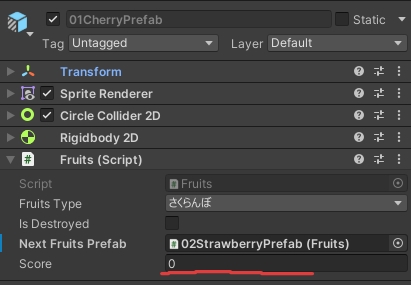
フルーツ | 得点 |
---|---|
さくらんぼ | 0 |
いちご | 1 |
ぶどう | 3 |
みかん | 6 |
柿 | 10 |
りんご | 15 |
なし(梨) | 21 |
もも | 28 |
パイナップル | 36 |
メロン | 45 |
スイカ | 55 |
スコアを表示する処理を追加
得点を得る部分を作りましたので、あとはスコア表示を反映するところを作りましょう。
UIを作成
UIは画面左上にImageで枠を作り、その中にTextMeshProでスコアを表記できるようにしましょう。バランス的には下のようなものを用意してください
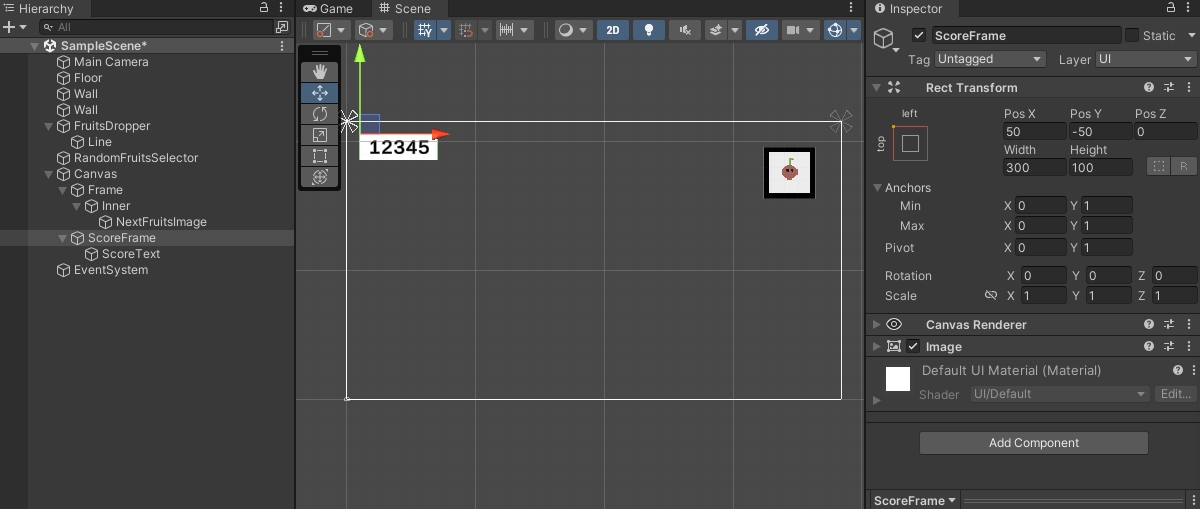
- ScoreFrame用のImageを追加(RectTransformの設定は上図参照)
- ScoreFrameの下にTextMeshProを追加
- 名前を変更:ScoreText
- フォントサイズ:75
- 太字にしています
- 右詰めにしておくと見やすいかも
スコア表示用のスクリプトを作成
スクリプトでは、先程作成したTextMeshProの更新を行います。スコアは集計しているところがないので、これから作成するスクリプトでスコア自体も集計したいと思います。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using TMPro;
public class DisplayScore : MonoBehaviour
{
public TextMeshProUGUI scoreText;
private int score = 0;
private void Start()
{
Fruits.OnScoreAdded.AddListener(AddScore);
AddScore(0);
}
private void AddScore(int score)
{
this.score += score;
scoreText.text = this.score.ToString();
}
}
StartメソッドのAddScore(0)は表示のリセットです。
インスペクターのセット
スクリプトはScoreFrameにセットしましょう。インスペクターには子供にあるはずのScoreTextをセット。
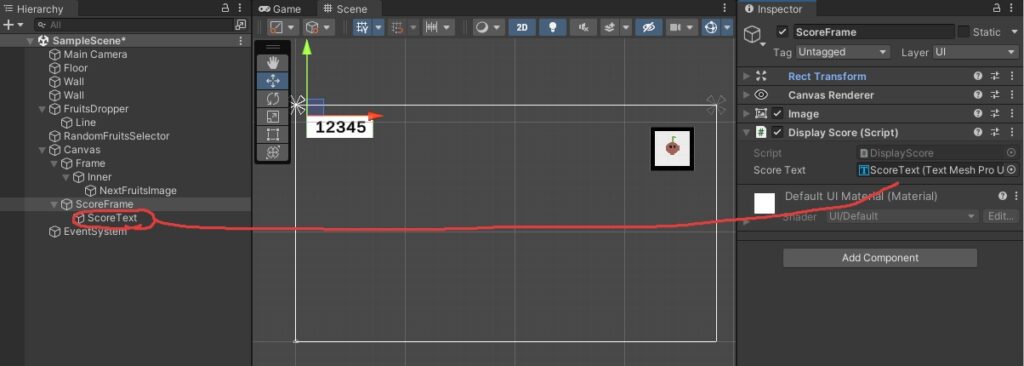
動作確認
目指せ3000点!(本家より大きさのバランスが優しいのでわりと簡単に行けるかも)
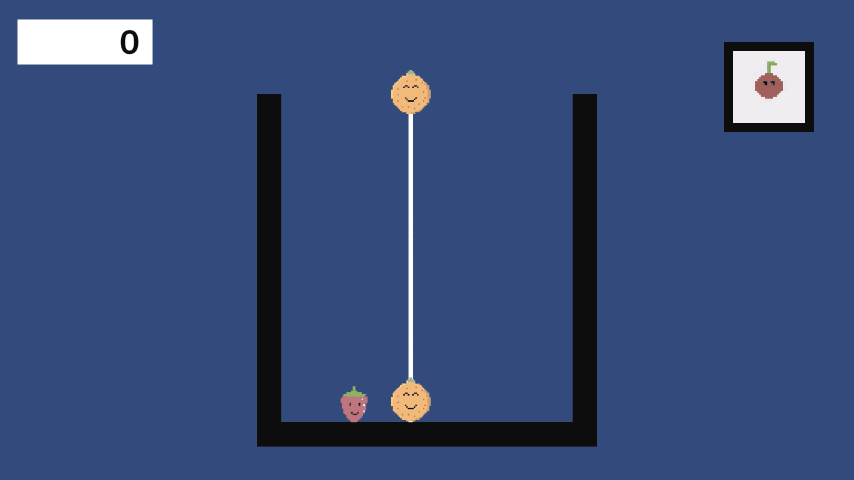
次のチャプター

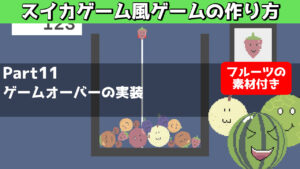
SG-ゲームオーバーの実装!part11
現在の状態では一生ゲームが遊べます。ゲームが終了するための条件を追加して、このゲームを完成させましょう。 【ゲーム終了判定をとる】 ゲーム終了の判定方法ですが…
コメント
コメント一覧 (1件)
[…] SG-スコアアップと表示part10 […]